Casting in Unreal Engine 5 with C++
Real quick let's go over casting in UE5 with C++
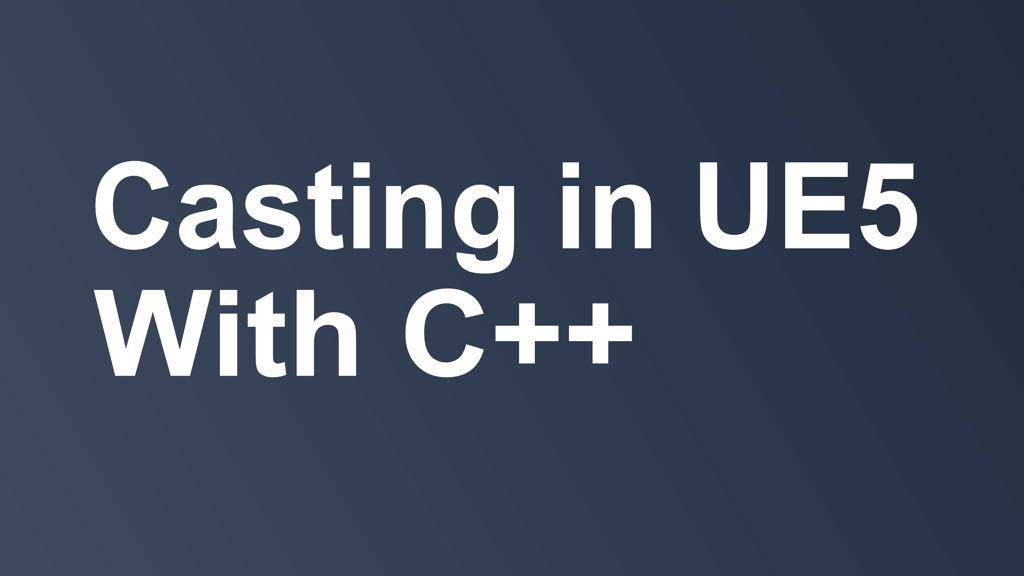
Game Engine
Unreal Engine 5.5.1
Casting is when you convert data or a variable to another type. We typically use casting in Unreal to get the type of actors where we need to check the type before proceeding. Common use case are when you need to check the type of a overlapping actor, hit actor, player character, etc. to then act upon.
Below is a quick example where we're casting to the third person character and then first checking if MyCharacter
is valid before calling MyCharacter
class function.
AActor* MyActor = GetWorld()->GetFirstPlayerController()->GetPawn();
ATP_ThirdPersonCharacter* MyCharacter = Cast<ATP_ThirdPersonCharacter>(MyActor);
if (MyCharacter != nullptr)
{
// we can now act upon on MyCharacter variable
// MyCharacter->CharacterFunction();
}
else
{
// Cast failed
}
``
Below is another example inside an overlap event. We cast to the OtherActor
in the function's overlap params that has entered the BoxComponent
. Check if the the cast was successful before proceeding.
void AMyCast::OnBeginOverlap(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor != nullptr && OtherActor != this)
{
ATP_FirstPersonCharacter* MyFirstPersonCharacter = Cast<ATP_FirstPersonCharacter>(OtherActor);
if (MyFirstPersonCharacter)
{
// MyFirstPersonCharacter->MyFunction();
}
}
}
Another option is to use CastChecked
. Only use CastChecked
if you're certain of they type and the cast will succeed. This can be great for debugging. use CastChecked
with caution.
APawn* MyPawn = GetWorld()->GetFirstPlayerController()->GetPawn();
ATP_ThirdPersonCharacter* MyCharacterChecked = CastChecked<ATP_ThirdPersonCharacter>(MyPawn);
// MyCharacterChecked->CharacterFunction();
Cast
is very commonly used, we'll likely see in all the projects we work in. Hopefully this post helped a lil bit.
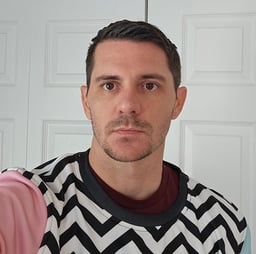
Harrison McGuire