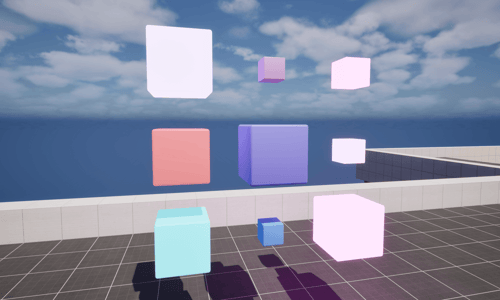
Using Custom Primitive Data to Change Material Parameters in Unreal Engine 5 Using C++
Another great way to change material properties is through using custom primitive data.
Another great way to change material properties is through using custom primitive data.
Let's use the quadratic formula to aim ahead and shoot a moving target.
Let's use UE5's ApplyDamage function to broadcast a damage event to receiving actors.
UE5 has a few different techniques of how to handle damage and one of them is that actor's OnTakeAnyDamage delegate.
Setting the tick interval in UE5 can be a great way to limit tick iterations
Certain delegate types have access to a static CreateLambda function that can be fun to use.
UE5 has some great preprocessor directives already established in the code base we can leverage.
Let's a create basic plugin for Unreal Engine 5 so all our projects can share resources.
Real quick let's have some fun setting the gravity scale in the movement component
Let's add coyote time in UE5 to our third person character