Create a Custom Plugin in Unreal Engine 5
Let's a create basic plugin for Unreal Engine 5 so all our projects can share resources.
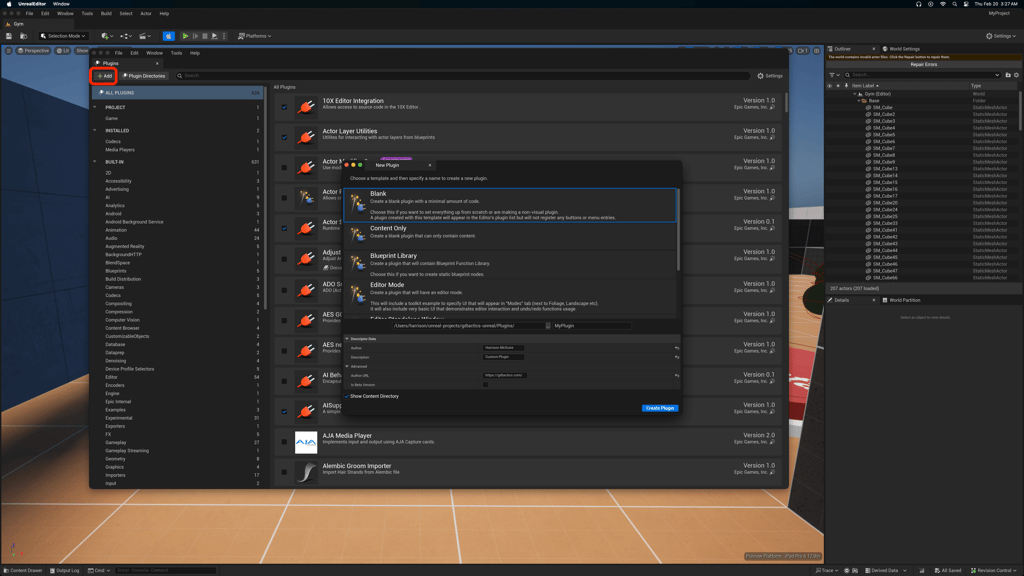
Game Engine
Unreal Engine 5.5.3
IDE
Rider 2024.3.5
Project Name
MyProject
OS
macOS Sequoia 15.3.1
Let's create a plugin. A custom plugin can be helpful when needing to share resources amongst different projects.
First, add a new plugin. Either add the new plugin via the editor or IDE. Rider had the option to add the plugin to the project's uproject
plugins list. The modules might have to rebuild on the next start up. Add a new plugin however you feel comfortable, I'm choosing a blank plugin for simplicity.
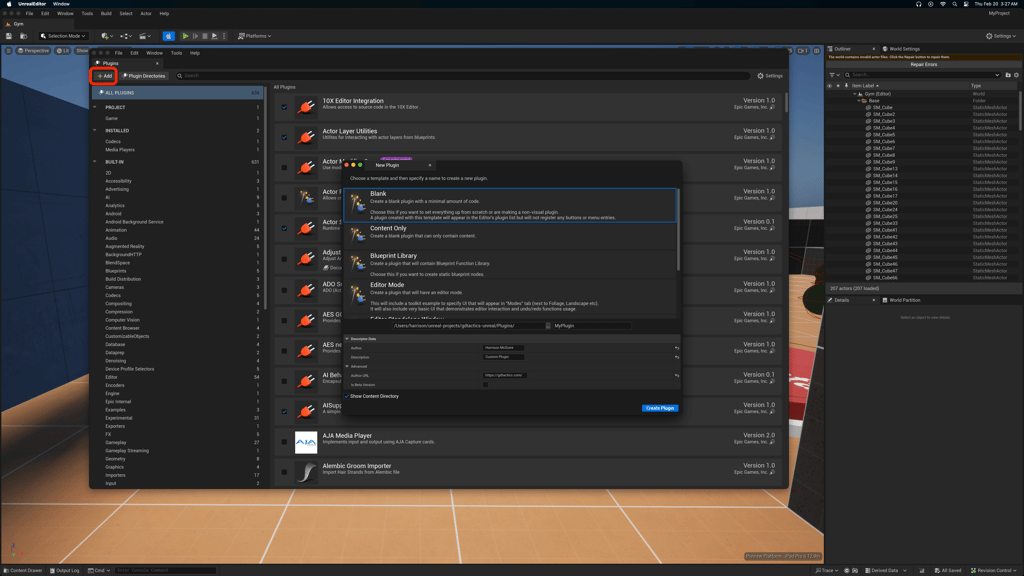
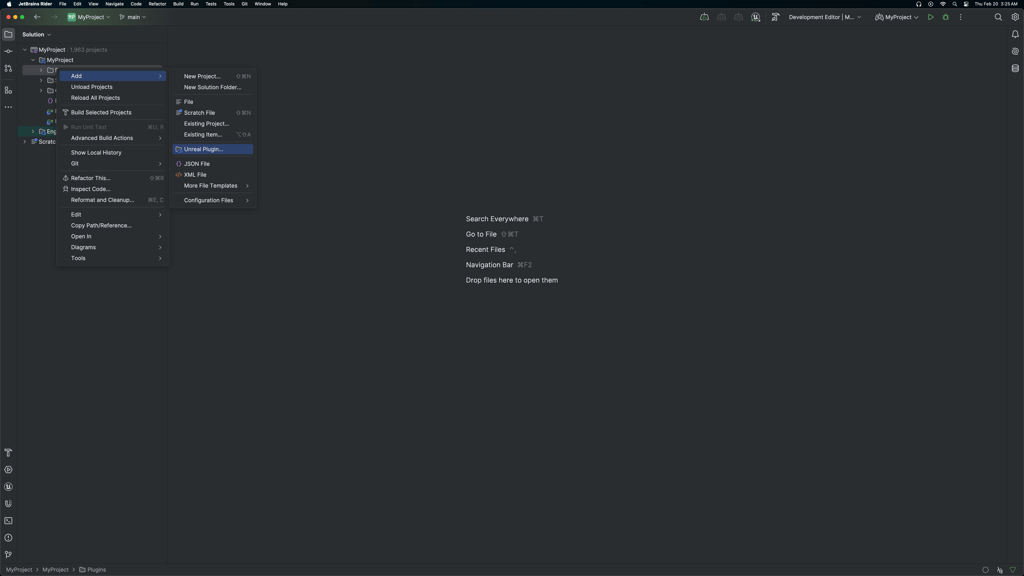
With this example we created a Blank plugin, we've could have just as easily created a Blueprint Library Plugin to speed up the process, but I wanted to take it step by step to see the pieces come together.
In this example I'm creating a Blueprint Function Library plugin, so we'll need to create a new Blueprint Library class that lives in the plugin. We can do that via the IDE.
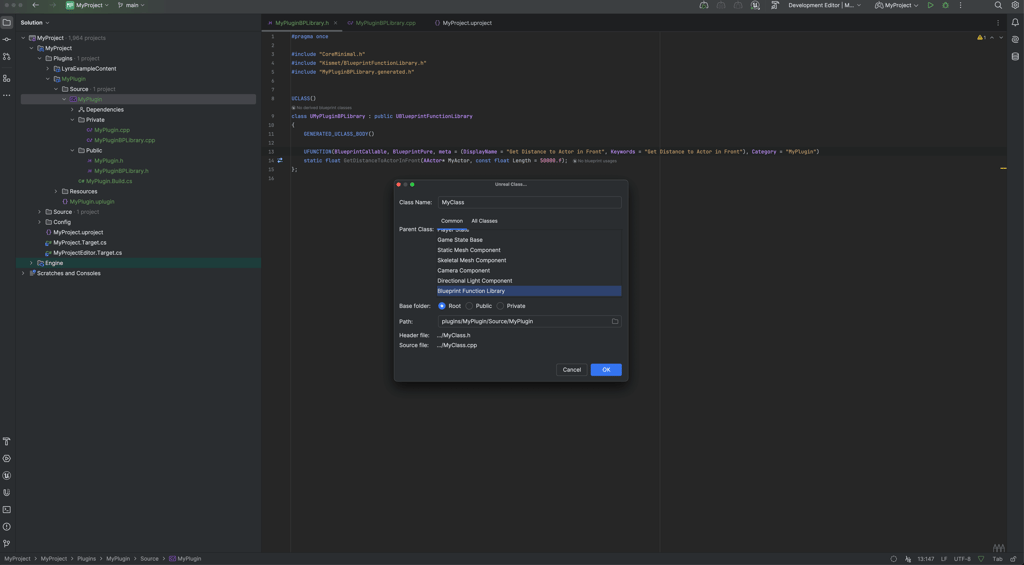
When editing the Blueprint Library, in my experience, I had to always restart the editor to see the changes take effect.
To keep things simple I wanted to create a basic function that returns the distance to an actor using a line trace. Doing this will hopefully reduce some boilerplate future code. The meta
options inside the UFUNCTION
macro are awesome because we add DisplayName
and Keywords
to our function for ease of use in Blueprints.
MyPluginBFLibrary.h
#pragma once
#include "CoreMinimal.h"
#include "Kismet/BlueprintFunctionLibrary.h"
#include "MyPluginBFLibrary.generated.h"
UCLASS()
class UMyPluginBFLibrary : public UBlueprintFunctionLibrary
{
GENERATED_UCLASS_BODY()
UFUNCTION(BlueprintCallable, BlueprintPure, meta = (DisplayName = "Get Distance to Actor in Front", Keywords = "Get Distance to Actor in Front"), Category = "MyPlugin")
static float GetDistanceToActorInFront(AActor* MyActor, const float TraceLength = 50000.f);
};
MyPluginBFLibrary.cpp
#include "MyPluginBFLibrary.h"
UMyPluginBFLibrary::UMyPluginBFLibrary(const FObjectInitializer& ObjectInitializer)
: Super(ObjectInitializer)
{
}
float UMyPluginBFLibrary::GetDistanceToActorInFront(AActor* MyActor, const float TraceLength)
{
if (MyActor == nullptr) { return 0.f; }
UWorld* const World = MyActor->GetWorld();
FVector StartLocation = MyActor->GetActorLocation();
FVector TraceDistance = MyActor->GetActorForwardVector() * TraceLength;
FVector EndLocation = StartLocation + TraceDistance;
FHitResult OutHit;
bool bHit = World->LineTraceSingleByChannel(OutHit, StartLocation, EndLocation, ECC_Visibility);
if (bHit)
{
return OutHit.Distance;
}
else
{
return 0.f;
}
}
After we rebuild we now have a custom plugin that we can use in our Blueprints.
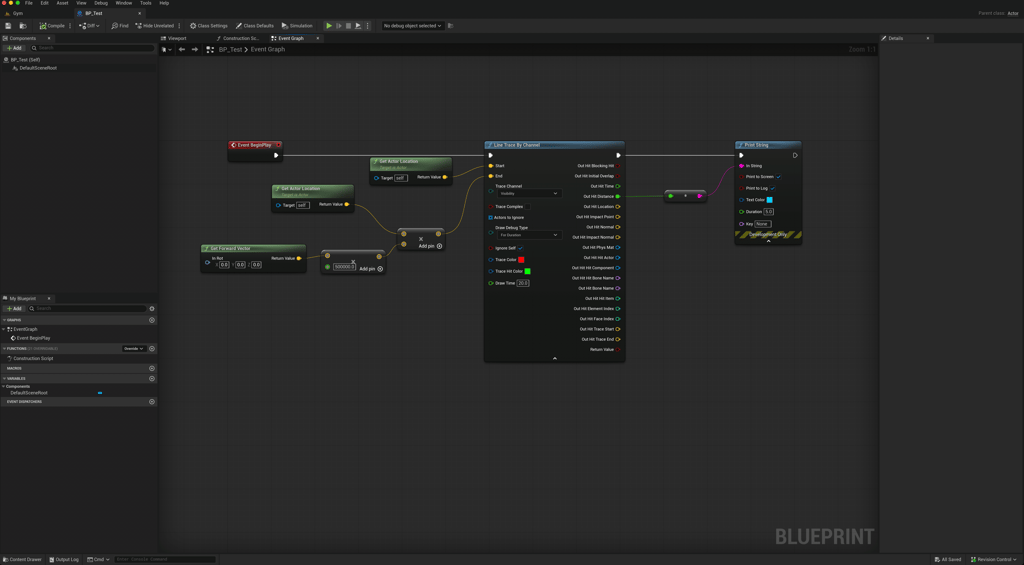
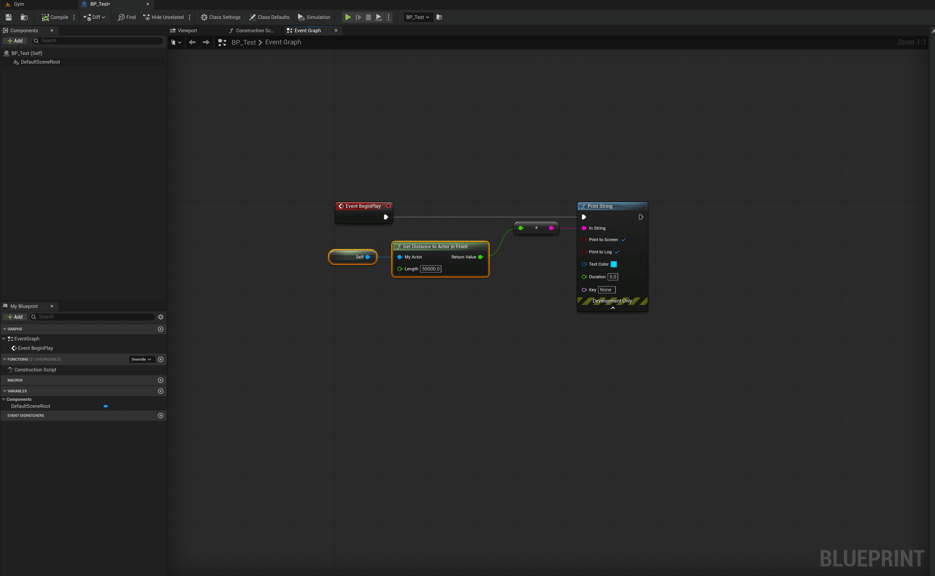
I hope this helped. I look forward to extending this plugin and adding helpful utilities that I can use across projects. It'll be fun to try new things while keeping functions isolated to their own plugin container.