Forward Declarations in Unreal Engine 5
Using forward declarations in your header files allows you to reference classes not yet loaded or included.
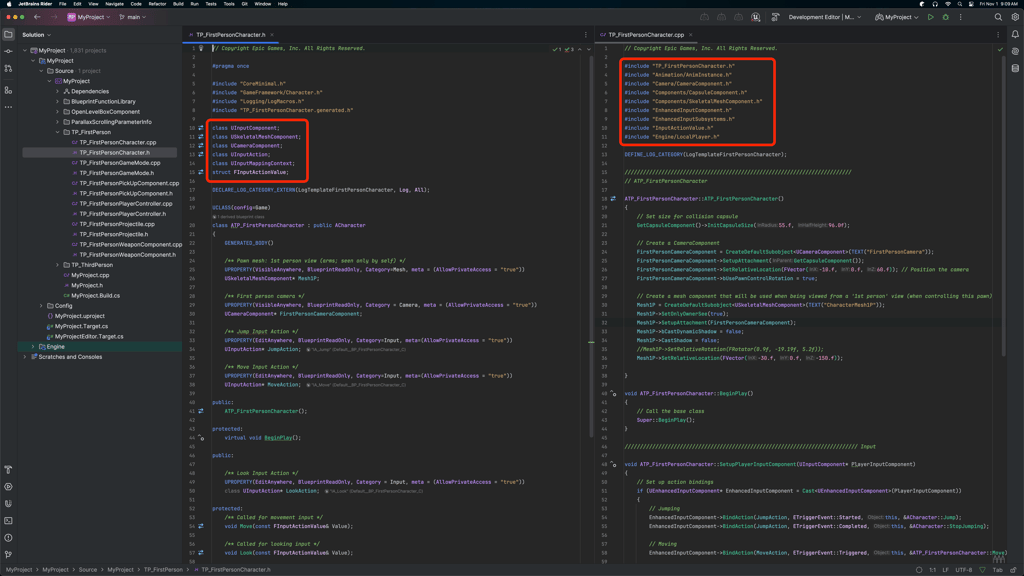
Software Version: Unreal Engine 5.4.4
Forward declaration is when you declare something that will be loaded or defined later in the program. This isn't anything new to most C++ developers, but if you're coming from another language or engine this is great to know. Forward declarations help eliminate circular dependencies that can cause issues in the program.
The First Person Shooter starter project has an awesome example of forward declarations. Inside the FirstPersonCharacter.h
file they declare many classes near the beginning that are not included at the top of the file. The classes are referenced in the header file, but the actual code is included/imported later in the .cpp
file.
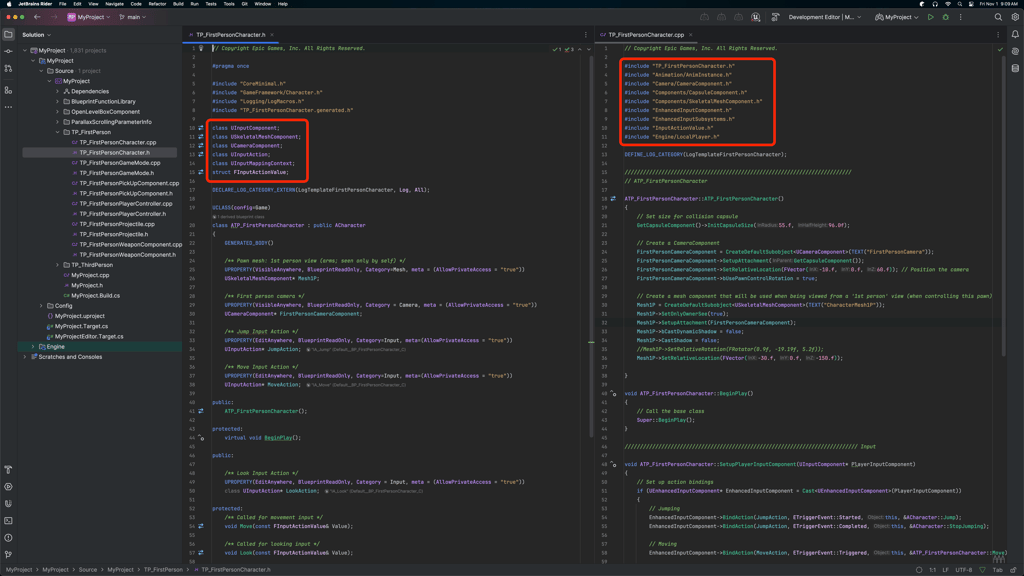
For example inside TP_FirstPersonCharacter.h
the USkeletalMeshComponent
is declared on line 11 and then used on lines 26 and 66. The actual component is then included inside TP_FirstPersonCharacter.cpp
on line 7.
TP_FirstPersonCharacter.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
#include "Logging/LogMacros.h"
#include "TP_FirstPersonCharacter.generated.h"
class UInputComponent;
class USkeletalMeshComponent;
class UCameraComponent;
class UInputAction;
class UInputMappingContext;
...
/** Pawn mesh: 1st person view (arms; seen only by self) */
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category=Mesh, meta = (AllowPrivateAccess = "true"))
USkeletalMeshComponent* Mesh1P;
...
/** Returns Mesh1P subobject **/
USkeletalMeshComponent* GetMesh1P() const { return Mesh1P; }
...
TP_FirstPersonCharacter.cpp
#include "TP_FirstPersonCharacter.h"
#include "Animation/AnimInstance.h"
#include "Camera/CameraComponent.h"
#include "Components/CapsuleComponent.h"
#include "Components/SkeletalMeshComponent.h"
#include "EnhancedInputComponent.h"
#include "EnhancedInputSubsystems.h"
#include "InputActionValue.h"
#include "Engine/LocalPlayer.h"
...
Forward declaration allows the .h
file to be included in many other .cpp
files without causing a circular dependency issue.
Again, probably old news to a lot of people, but I had to learn the hard way coming other languages.
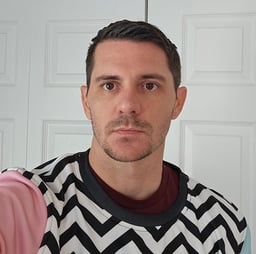
Harrison McGuire