Going Over the Different Ways to Create an FTransform Value with C++ in UE5
There are a few techniques to create an FTransform value in Unreal, let's go over them real quick.
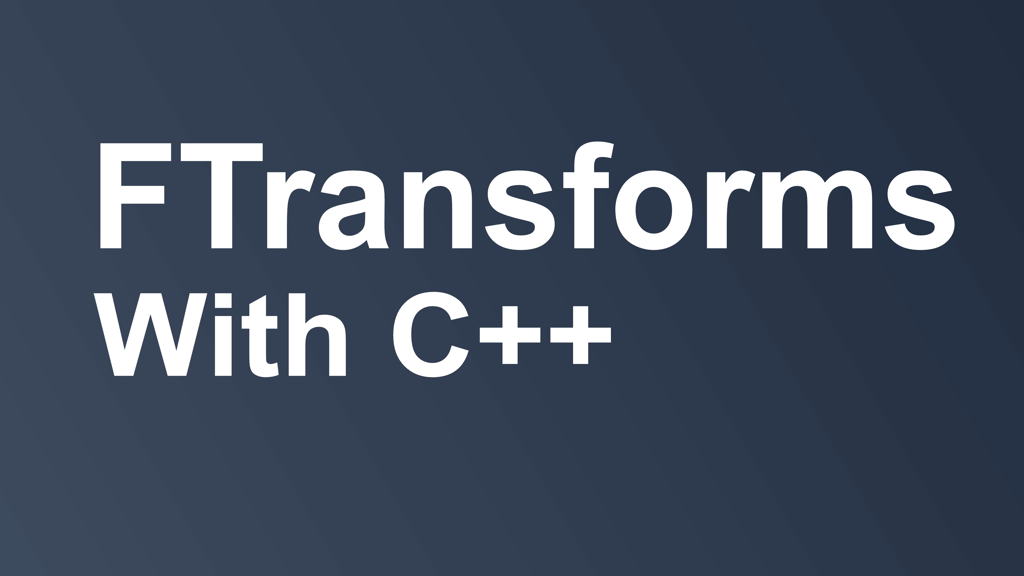
Game Engine
Unreal Engine 5.5.1
There are a few different ways to set FTransform
values with C++ in Unreal Engine 5. The basic way is on init
when the variable is declared to set the location/translation or the rotation of the variable. The constructor will understand the type of argument being passed and set defaults for the remaining values.
// sets location, rotation defaults to FQuat::Identity and scale defaults to FVector(1)
FTransform MyTransform1(FVector::ZeroVector);
// sets rotation, location defaults to FVector::ZeroVector and scale defaults to FVector(1)
FTransform MyTransform2(FQuat::Identity);
Alternatively, we can set all three values of the FTransform
value. When we do this, the rotation value comes first, followed by the location/translation then scale.
// set all three parameters. Rotation as a Quaternion comes first.
FTransform MyTransform3(FQuat::Identity, FVector::ZeroVector, FVector(1));
FTransform
values also come with their setters and getters. We set each value of an FTransform
individually with its corresponding setting function.
// set using FTransform setters
FTransform MyTransformSet;
MyTransformSet.SetLocation(GetActorLocation());
MyTransformSet.SetRotation(GetActorRotation().Quaternion());
MyTransformSet.SetScale3D(FVector(1, 1, 1));
Finally, the KismetMathLibrary
provides a MakeTransformFunction
function we can use. This function takes an FVector
for location as the first parameter followed by the rotation and scale. UKismetMathLibrary::MakeTransform
simply just returns return FTransform(Rotation, Translation, Scale);
so it might be a little extra overhead not needed.
// create a transform using the UKismetMathLibrary library.
FVector MyLocation = GetActorLocation();
FRotator MyRotator = GetActorRotation();
FVector MyScale = FVector(1);
FTransform MyTransformLib = UKismetMathLibrary::MakeTransform(MyLocation, MyRotator, MyScale);
Use whatever technique works best for you or your situation. I hope this helped a little bit.
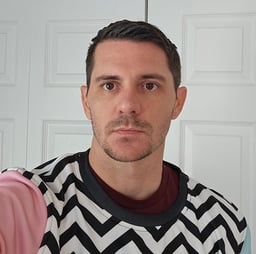
Harrison McGuire