Setting Tick Interval in Unreal Engine 5 via Blueprints and C++
Setting the tick interval in UE5 can be a great way to limit tick iterations
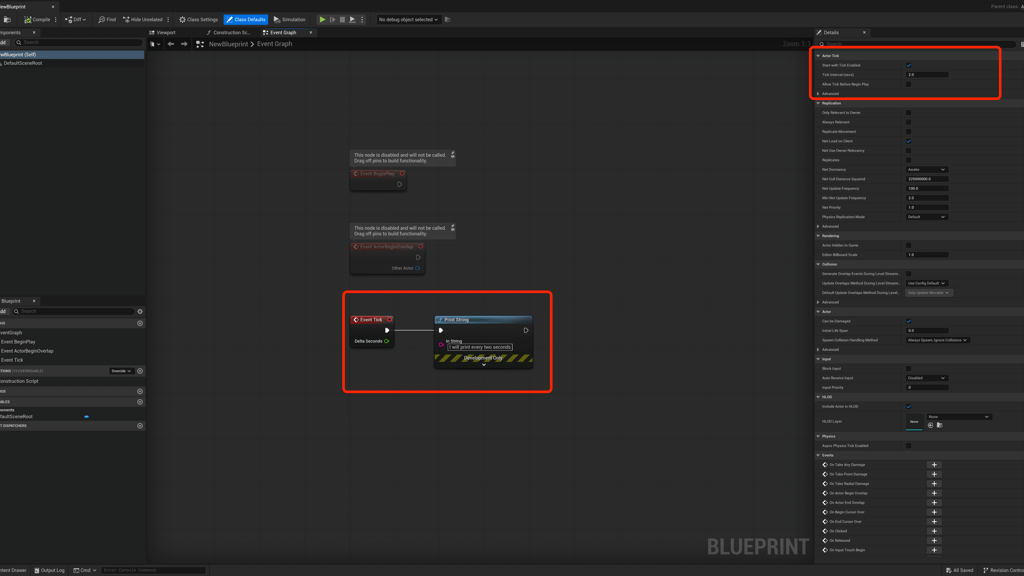
Game Engine
Unreal Engine 5.5.3
IDE
Rider 2024.3.6
Project Name
MyProject
OS
macOS Sequoia 15.3.1
There's always a lot of talk about the side effects of tick
and how non-performant it can be, which is true when abused, but in some situations we utilize limiting tick intervals to reduce the load.
Tick, by definition, runs every frame, but sometimes running every half second would suffice for our use case. Typically we would create timer to handle interval calls, however, alternatively we can set the tick interval for quick iteration. For example, in the Blueprint editor for a new actor we can set the Tick Interval in the details panel to be set to two seconds and then whatever runs in the Tick
function will be called every two seconds.
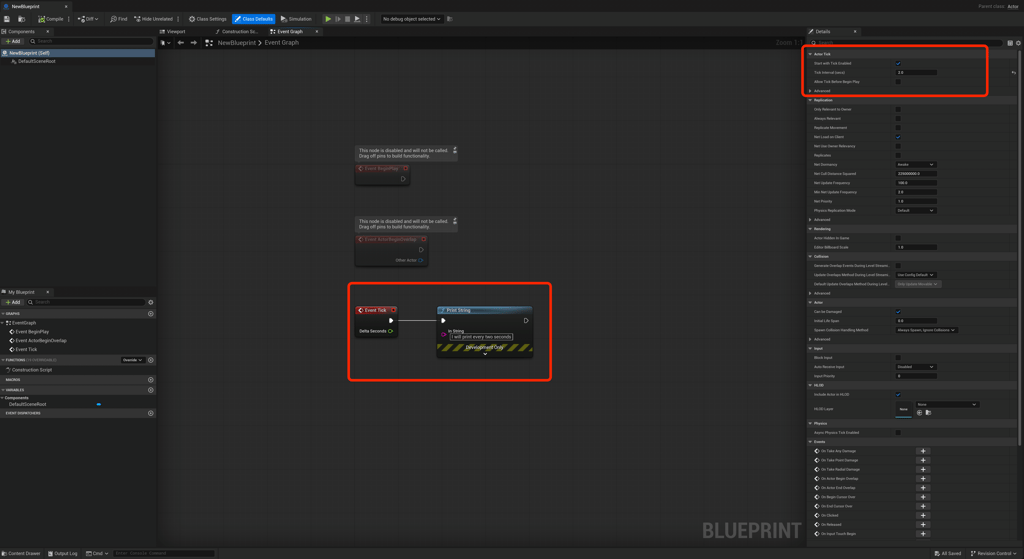
We can also do this in C++ with the actor's SetActorTickInterval(float TickInterval)
function. For example in the actor's constructor or BeginPlay
function (or wherever is best for your use case), we can call SetActorTickInterval
. Below I'll set it to run every tenth of second so it'll be called 10 times every second rather than 60 times, or 90 times, or 120 or etc...depending on how many frames the game is pumping out.
AMyActor::AMyActor()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
SetActorTickInterval(0.1f);
}
After discovering SetActorTickInterval
I was pretty delighted to know that there is a very simple way to create timer functions without having to use a handler and bind function. This will also help with optimization for those times when you just need to put logic somewhere, but don't need it every frame.