Using C++ to Bind to a Delegate in Unreal Engine 5
Quick simple example of how to use BindRaw to bind to a delegate created in another class
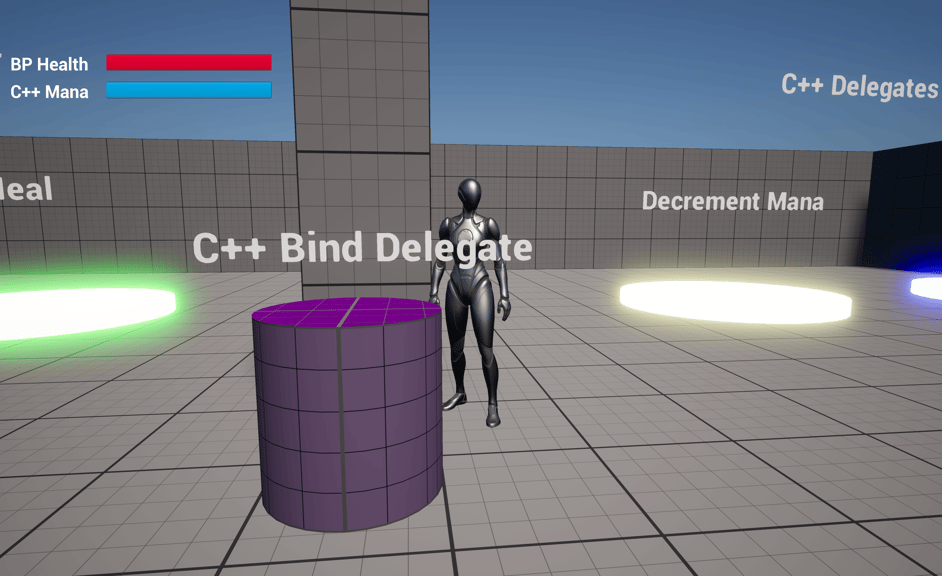
Software Versions: Unreal Engine 5.5.0 | Rider 2024.2.7
Project Name: MyProject
Building off a couple of previous posts (Dispatchers and Delegates) I wanted to go through Epic's documentation and bind a delegate with C++ using their LogWriter
example.
I started by creating a non-UObject
class, so just a regular C++ class, and called it FLogWriter
. The class is very simple, it declares a one parameter delegate and one WriteLog
function that accepts one FString
parameter. The WriteLog
function simply writes to the console.
FLogWriter.h
#pragma once
DECLARE_DELEGATE_OneParam(FStringDelegate, FString);
class FLogWriter
{
public:
void WriteToLog(FString MyText);
};
FLogWriter.cpp
#include "FLogWriter.h"
void FLogWriter::WriteToLog(FString MyText)
{
UE_LOG(LogTemp, Warning, TEXT("Log Writer Delegate Text: %s"), *MyText);
}
Next, I created a new actor class and called it MyDelegateBindActor
. The header file imports the FLogWriter
class to declare a delegate signature. Then inside the constructor we declare a shared reference, TSharedRef
, to the FLogWriter
class and use BindRaw
to bind to the WriteToLog
function.
Finally, for a simple example in BeginPlay
we use ExecuteIfBound
to call the function while passing in an argument.
When testing I Couldn't get BindSP
to work with IsBound
or ExecuteIfBound
. So Instead I used BindRaw
MyDelegateBindActor.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "FLogWriter.h"
#include "MyDelegateBindActor.generated.h"
UCLASS()
class MYPROJECT_API AMyDelegateBindActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AMyDelegateBindActor();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
FStringDelegate WriteToLogDelegate;
};
MyDelegateBindActor.cpp
#include "MyDelegateBindActor.h"
// Sets default values
AMyDelegateBindActor::AMyDelegateBindActor()
{
TSharedRef<FLogWriter> LogWriter(new FLogWriter());
WriteToLogDelegate.BindRaw(&LogWriter.Get(), &FLogWriter::WriteToLog);
}
// Called when the game starts or when spawned
void AMyDelegateBindActor::BeginPlay()
{
Super::BeginPlay();
WriteToLogDelegate.ExecuteIfBound(TEXT("Delegate Exectuing if Bound"));
}
With the new class compiled I create a new BP actor that inherits from MyDelegateBindActor
and added a basic cylinder mesh and dragged it into the scene. On BeginPlay
a message appears in the console.
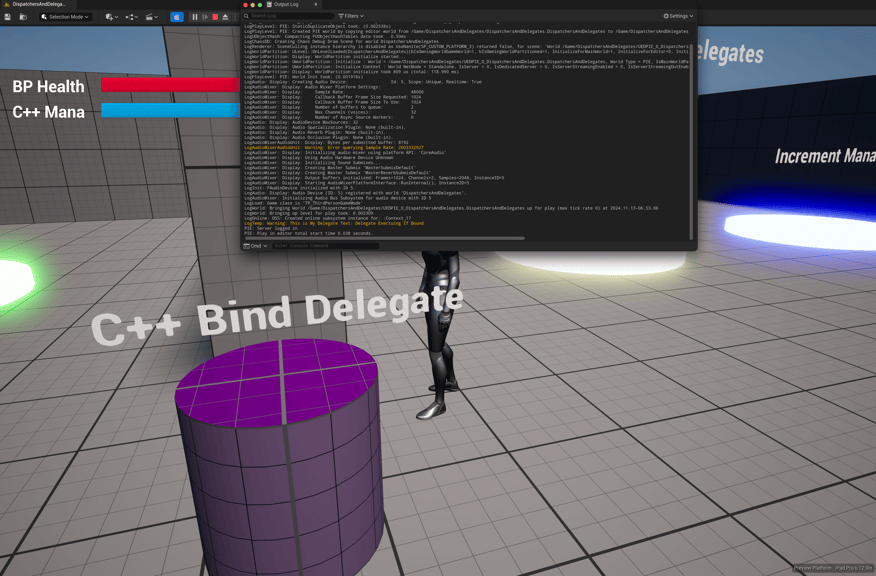
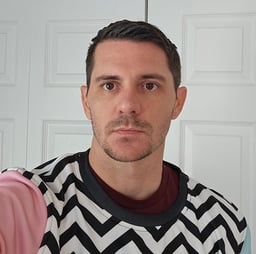
Harrison McGuire