Using the OnTakeAnyDamage Delegate to Receive Damage in C++
UE5 has a few different techniques of how to handle damage and one of them is that actor's OnTakeAnyDamage delegate.
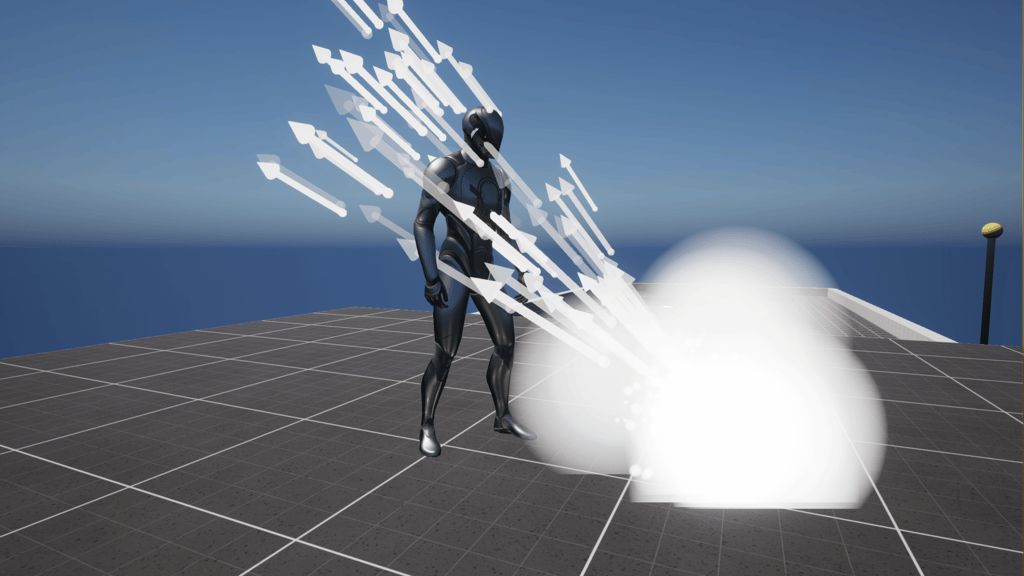
Game Engine
Unreal Engine 5.5.3
IDE
Rider 2024.3.6
Project Name
MyProject
OS
macOS Sequoia 15.3.1
In Unreal every actor can take damage. There is point damage and radial damage, for this example I want to focus on OnTakeAnyDamage
to account for any damage type. Any actor can take advantage of the OnTakeAnyDamage
delegate to perform logic when another actor uses UGameplayStatics::ApplyDamage
.
We can use the OnTakeAnyDamage
delegate by can using the delegate's AddDynamic
macro inside the actor's constructor. For example, similar to overlap events, we can do something like the below snippet to bind a damage event to OnTakeAnyDamage
.
MyCharacter.h (example)
...
float Health = 100.f;
UFUNCTION()
void MyDamageFunction(AActor* DamagedActor, float Damage, const class UDamageType* DamageType, class AController* InstigatedBy, AActor* DamageCauser);
MyCharacter.cpp (example)
AMyCharacter::AMyCharacter()
{
...
OnTakeAnyDamage.AddDynamic(this, &AMyCharacter::MyDamageFunction);
}
void AMyCharacter::MyDamageFunction(AActor* DamagedActor, float Damage, const class UDamageType* DamageType, class AController* InstigatedBy, AActor* DamageCauser)
{
Health -= Damage;
if (Health <= 0.f)
{
Destroy();
}
}
To trigger the event another actor can use ApplyDamage
. The ApplyDamage post can bee seen here. Hopefully this helped.